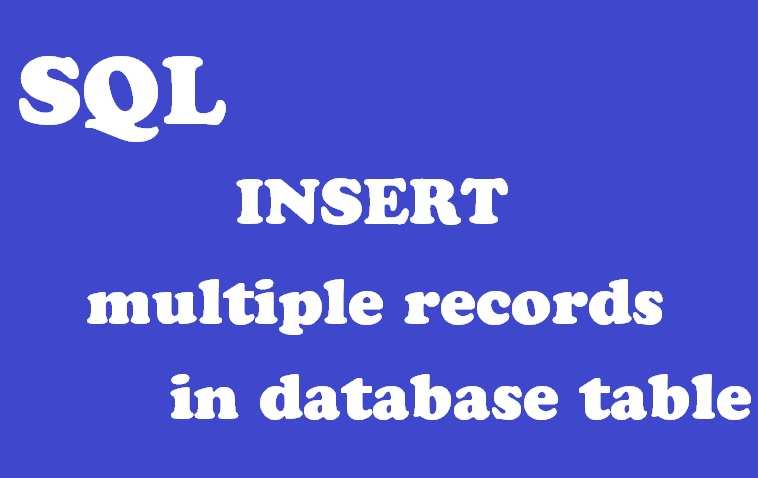
In SQL, the INSERT query is used to insert data into the database. It is a DML(Data Manipulation Language) which is one of the most common queries in SQL to manipulate table data. If you want to learn more about SQL, here is the best SQL Tutorial you can find helpful.
In this post, we will see how to insert multiple-row data in the table of the database using the INSERT query.
We will first create a database table from scratch and then we will insert multiple rows of data afterward.
Creating database table using SQL query
Let’s create a table named ’employee’
create table employee(
id int,
name varchar(10)
address varchar(255)
);
id | name | address |
Inserting a single record of data in the table
Let’s first add a single record in the above empty table:
INSERT INTO employee (id, name, address)
VALUES (1, 'Tom', 'New York');
id | name | address |
01 | Tom | New York |
Inserting multiple records in the table using SQL query
There are multiple ways one can insert multiple rows of records in a database table using the SQL query. We will talk about two of them.
1st way) Using Multiple INSERT Statements
One way to insert multiple rows is to use a separate INSERT
statement for each row:
INSERT INTO employee(id, name, address) VALUES (2, 'Brad', 'Chicago');
INSERT INTO employee(id, name, address) VALUES (3, 'Vin', 'Los Angeles');
INSERT INTO employee(id, name, address) VALUES (4, 'Will', 'Austin');
id | name | address |
1 | Tom | New York |
2 | Brad | Chicago |
3 | Vin | Los Angeles |
4 | Will | Austin |
2nd way) Provide All Data in the VALUES Clause
INSERT INTO employee(id, name, address)
VALUES
(2, 'Brad', 'Chicago'),
(3, 'Vin', 'Los Angeles'),
(4, 'Will', 'Austin')
id | name | address |
1 | Tom | New York |
2 | Brad | Chicago |
3 | Vin | Los Angeles |
4 | Will | Austin |