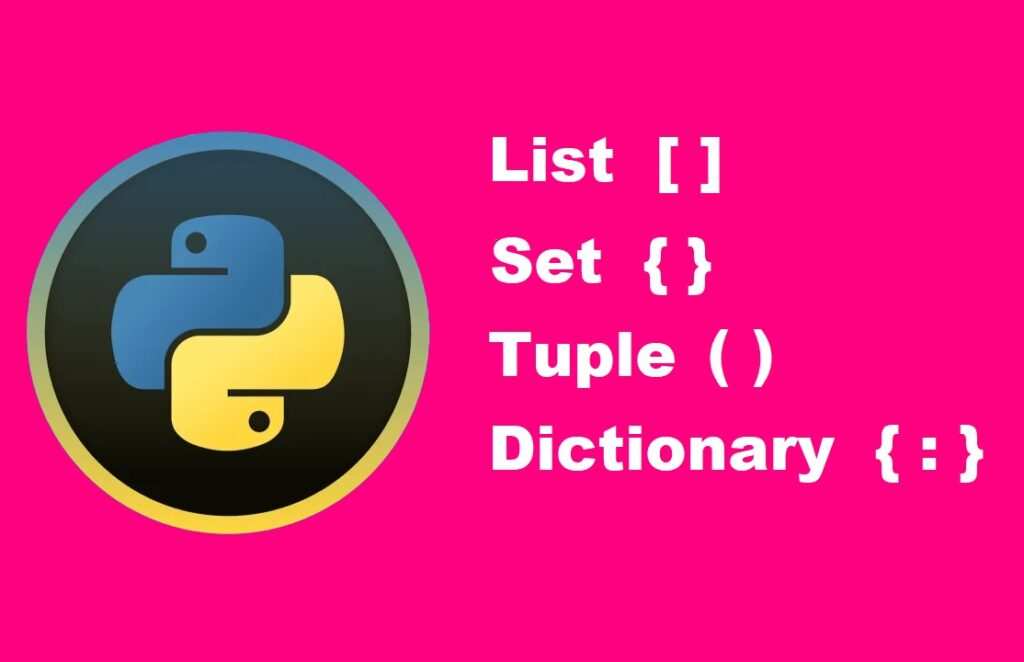
What is Data Structure?
Data Structure is a data storage format that allows for the efficient access and manipulation of the stored data. If the data is stored in a structured format, then it would be easy for a programming language to deal with it like accessing, searching, manipulating, etc.
In Python, the basic data structures include lists, sets, tuples, and dictionaries. Each of the data structures is unique in its own way. We will see all of them one by one.
Different Data Structures in Python
List
Lists are one of the simple and most commonly used data structures provided in python. It can store multiple data types at the same time like string, int, boolean, etc, and hence it is called heterogeneous.
Lists are mutable which means the data inside the list can be altered. Lists contain ordered data and can also contain duplicate values.
- Heterogeneous
- Mutable
- Ordered
- Can have duplicate value
Eg: mylist = [45, 37, ‘ABC’, 56, 89]
Tuple
Tuples are just like lists except that they are immutable which means data inside a tuple can only be accessed but cannot be altered.
- Heterogeneous
- Immutable
- Ordered
- Can have duplicate values
Eg: mytuple = (25, 30, 35, 40)
Set
Set is another data structure that holds a collection of unordered, iterable, and mutable data. But it only contains unique elements. They can contain multiple data types and hence are heterogeneous.
- Heterogeneous
- Mutable
- Unordered
Eg: myset = {“US”, “UK”, “Canada”}
Dictionary
It is slightly different from other data structures in which data are stored in the form of key-value pairs. Keys inside the dictionaries should be unique whereas their value(s) need not necessarily be unique. Any data inside the dictionary can be accessed through its key.
- Heterogeneous
- Mutable
- Unordered before Python v3.7, Ordered in Python v3.7 or more
- Do not allow duplicate values
Eg: myDictionary = {“brand”: “Ford”, “model”: “Mustang”, “year”: 1964}
Working with Lists
There are lots of operations you can do with a list. As told above, a list is mutable, so you not just can access its data but also manipulate it. Let’s see it:
a) Creating a list and printing its data
mylist = ["Python", "Javascript", "HTML"]
print(mylist)
#Output: ["Python", "Javascript", "HTML"]
mylist = ["Python", "Javascript", "HTML", "Python", "HTML"]
print(mylist)
#Output: ["Python", "Javascript", "HTML", "Python", "HTML"]
# As you can see it allows duplicate values
b) List length
To determine how many items a list has, the len()
function is used.
mylist = ["Audi", "Mercedes", "Rolls Royce"]
print(len(mylist))
#Output: 3
c) Accessing Items on a list
As lists are iterable, we can access any element(s) of a list through its index value. In Python, counting starts from 0. So to get the first element of the list we use the index 0 so on and so forth.
list = ["HTML", 30, "Days", "Learning"]
print(list[0])
#Output: HTML
print(list[1])
#Output: 30
print(list[3])
#Output: Learning
Accessing list items in a custom way
Getting from the last:
mylist = ["US", "Canada", "UK", "Mexico"]
print(mylist[-1])
#Output: Mexico
Will return the third, fourth, and fifth items:
mylist = ["US", "Canada", "UK", "Mexico", "France", "Germany", "Italy"]
print(mylist[2:5])
#Output: ["UK", "Mexico", "France"]
Returning items from the beginning to a certain point: This excludes the last point
mylist = ["US", "Canada", "UK", "Mexico", "France", "Germany", "Italy"]
print(mylist[:5])
#Output: ["US", "Canada", "UK", "Mexico", "France"]
Returning items from a certain point to the last:
mylist = ["US", "Canada", "UK", "Mexico", "France", "Germany", "Italy"]
print(mylist[3:])
#Output: ["Mexico", "France", "Germany", "Italy"]
d) Changing list items
Changing one value with another value:
mylist = ["US", "Canada", "UK"]
mylist[1] = "Mexico"
print(mylist)
#Output: ["US", "Mexico", "UK"]
Changing multiple values with multiple values:
mylist = ["US", "Canada", "UK", "Spain"]
mylist[1:3] = ["Mexico", "Brazil"]
print(mylist)
#Output: ["US", "Mexico", "Brazil", "Spain"]
Changing one value with two new values:
mylist = ["US", "Canada", "UK", "Spain"]
mylist[1:2] = ["Mexico", "Brazil"]
print(mylist)
#Output: ["US", "Mexico", "Brazil", "UK", "Spain"]
Changing two values with one new value:
mylist = ["US", "Canada", "UK", "Spain"]
mylist[1:3] = ["Mexico"]
print(mylist)
#Output: ["US", "Mexico", "Spain"]
e) Adding items to a list
We can add new items to a list either at the last or at any position. To insert an item in the last position of a list we use the ‘append()’ function and to insert a new item at a particular position in a list, we use the ‘insert()’ function. See the example below:
mylist = ["Python", "Java", "Javascript"]
mylist.append("ReactJS")
print(mylist)
#Output: ["Python", "Java", "Javascript", "ReactJS"]
mylist = ["Python", "Java", "Javascript"]
mylist.insert(1, "ReactJS")
print(mylist)
#Output: ["Python", "ReactJS", "Java", "Javascript"]
We can also combine two lists as can be seen in below code:
mylist = ["Python", "Java", "Javascript"]
yourlist = ["Laptop", "PC", "Tablet"]
mylist.extend(yourlist)
print(thislist)
#Output: ["Python", "Java", "Javascript", "Laptop", "PC", "Tablet"]
f) Removing an item from a list
Just like adding an item to a list, we can also remove it either from the last as by default or from a specified position. Items can be deleted either by using their name or through their index value. Let’s see the example below.
Remove
mylist = ["Python", "Java", "Javascript"]
mylist.remove("Java")
print(mylist)
#Output: ["Python", "Javascript"]
Pop
mylist = ["Python", "Java", "Javascript"]
mylist.pop()
print(mylist)
#Output: ["Python", "Java"]
#Pop removes an item from the last by default
mylist.pop(1)
print(mylist)
#Output: ["Python", "Javascript"]
Del
mylist = ["PC", "Mobile", "Tablet"]
del mylist[0]
print(mylist)
#Output: ["Mobile", "Tablet"]
del mylist
#Output: Will delete the whole list
g) Deleting the whole list or emptying its data
We can delete the whole list if needed or can just clear its data in python so easily. See the examples below:
mylist = ["PC", "Mobile", "Tablet"]
del mylist
#Output: Will delete the whole list
mylist = ["PC", "Mobile", "Tablet"]
mylist.clear()
print(mylist)
#Output: []
Read about Python find in list
Working with Sets
a) Creating a set and printing its data
myset = {'Python','Java','React'}
print(myset)
#Output: Python Java React
b) Accessing Items of a set
Since data is not in an ordered manner, you cannot access items in a set by referring to an index or a key. Instead, you can loop through the set items using a for
loop:
thisset = {'Laptop','PC','Tablet'}
for x in thisset:
print(x)
#Output: Laptop PC Tablet
c) Adding an item in a set
In Python we have ‘add()’ method to do the adding the item in the set:
thisset = {'Laptop','PC','Tablet'}
thisset.add('Smartphone')
print(thisset)
#Output: Laptop PC Tablet Smartphone
d) Adding two sets
We have ‘update()
‘ method in Python to add items from another set into the current set:
myset = {'US','UK','Canada'}
yourset = {'Australia','China'}
myset.update(yourset)
print(myset)
#Output: US UK Canada Australia China
e) Removing an item from a set
Using ‘remove()’ method:
thisset = {'Python', 'Java', 'MongoDB'}
thisset.remove('Java')
print(thisset)
#Output: Python MongoDB
Using ‘pop()’ method to remove a random item from a set:
myset = {'Apple','Microsoft','Google','Facebook'}
myset.pop()
print(myset)
f) Emptying all items from a set
To clear all the data stored in a set, we have the ‘clear()’ function in Python
myset = {'Apple','Microsoft','Google','Facebook'}
myset.clear()
print(myset)
#Output: set()
g) Deleting the whole set
The 'del'
keyword will delete the set completely:
myset = {'Apple','Microsoft','Google','Facebook'}
del myset
print(myset)
Working with Tuples
a) Creating a tuple and printing its data
thistuple = ('Javascript','Python','ReactJS')
print(thistuple)
#Output: Javascript Python ReactJS
b) Accessing tuple items
Since tuples are ordered, we can access any element of the tuple by calling its position:
thistuple = ('Jack','Tom','Riddle')
print(thistuple[1])
#Output: Tom
thistuple = ('Jack','Tom','Riddle')
print(thistuple[-1])
#Output: Riddle
c) Updating the value of the tuple
Tuples are immutable. Once a tuple is created, you cannot change its values. If you try to change or add or delete the data of a tuple, it will throw an error. You can only access the data and nothing else. But there is a way. You can first change a tuple into a list, then perform any operation of the list and then convert back the list into the tuple. Let’s see.
Updating the tuple:
First, convert the tuple into a list and then perform updating operation.
x = ('Laptop','PC','Tablet')
# Converting the tuple into a list
y = list(x)
y[1] = 'Smartphone'
# Converting the list back into the tuple
x = tuple(y)
print(x)
#Output: Laptop Smartphone Tablet
Adding an item in the tuple:
First, convert the tuple into a list and then perform adding operation.
mytuple = ('Apple','Microsoft','Google')
y = list(mytuple)
y.append('twitter')
mytuple = tuple(y)
#Output: Apple Microsoft Google Twitter
Removing an item from the tuple:
First, convert the tuple into a list and then perform the removing operation.
thistuple = ('Apple','Microsoft','Facebook')
y = list(thistuple)
y.remove('Microsoft')
thistuple = tuple(y)
#Output: Apple Facebook
Working with Dictionary
a) Creating and printing data from a dictionary
dicti = {
'brand': 'DELL',
'model': 'XPS 15',
'price': 2000
}
print(dicti)
#Output: {'brand' : 'DELL', 'model' : 'XPS 15', 'price' : 2000}
b) Accessing Items from a dictionary
Since data in a dictionary is stored in the form of keys and their associated values, we can access the value by referring its key:
dicti = {
'brand': 'DELL',
'model': 'XPS 15',
'price': 2000
}
x = dicti.get('price')
print(x)
#Output: 2000
c) Updating values of a dictionary
dicti = {
'brand': 'DELL',
'model': 'XPS 15',
'price': 2000
}
dicti['price'] = 3000
print(dicti)
#Output: {'brand': 'dell', 'model': 'xps 15', 'price': 3000}
d) Adding a new entry in a dictionary
We can easily add a new entry in a dictionary in Python. Just you have to provide the value to add with its key:
dicti = {
'brand': 'DELL',
'model': 'XPS 15',
'price': 2000
}
dicti['type'] = 'Office use'
print(dicti)
#Output: {'brand': 'dell', 'model': 'xps 15', 'price': 3000, 'type' : 'Office use'}
e) Removing an item from a dictionary
dicti = {
'brand': 'DELL',
'model': 'XPS 15',
'price': 2000
}
dicti.pop('model')
print(dicti)
#Output: {'brand' : 'DELL', 'price' : 2000}
f) Clearing the values of a dictionary
We can clear the whole dictionary data in Python easily with ‘clear()’ functions or can even delete the whole dictionary.
Clearing the values:
dicti = {
'brand': 'DELL',
'model': 'XPS 15',
'price': 2000
}
dicti.clear()
print(dicti)
#Output: {}
Deleting the whole dictionary:
dicti = {
'brand': 'DELL',
'model': 'XPS 15',
'price': 2000
}
del dicti
Related:
Python Basics
List, Set, Tuple, and Dictionary in Python
Python Reverse List items
Python Round() Function
Python Multiline comment
Power in Python | Python pow()
Python range() Function
Square Root in Python
Python for i in range