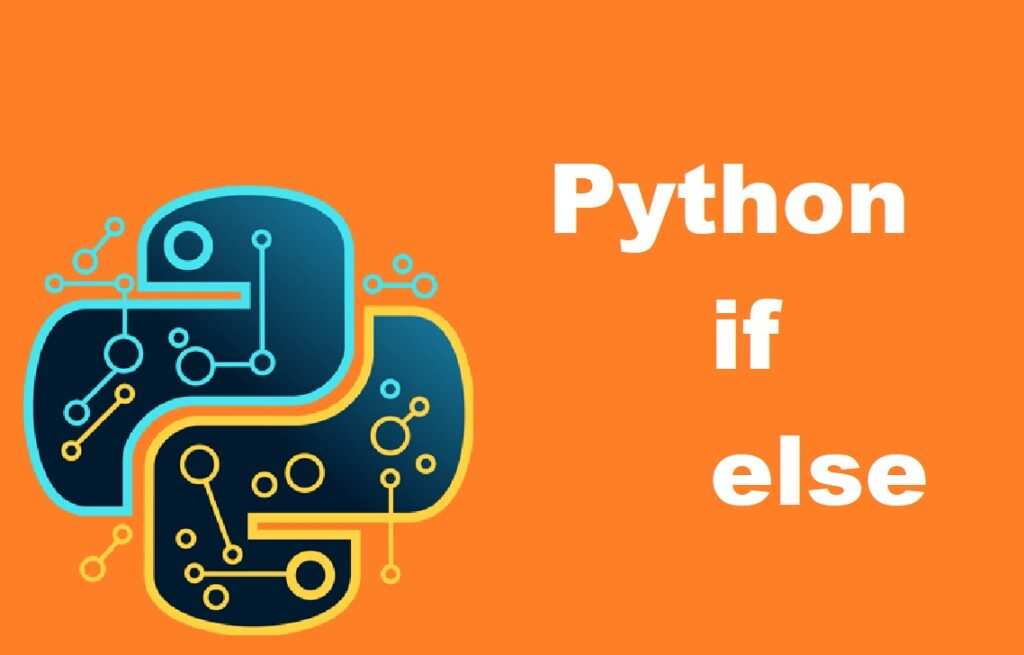
In Python, the if statement is used for conditional branching, allowing you to execute a block of code only if a certain condition is true. The basic syntax of the if statement in Python is as follows:
if condition:
# Code to be executed if the condition is True
Here, the condition is a boolean expression that evaluates to either True or False. If the condition is True, the code block indented underneath the if statement will be executed; otherwise, it will be skipped.
What are if, and else statements?
IF and else are the basic core concepts of any programming language which is used to perform certain tasks after the fulfillment of condition(s).
The piece of code first checks the statement, if it gets satisfied, the code will perform a certain fixed task, and if the condition fails to meet, the code will perform another fixed task. Let’s understand all these by an example.
Let’s suppose you have to greet your sir while he enters your classroom. If it is ‘Morning’, you have to say ‘Good Morning Sir!’. If it is ‘Afternoon’, you have to say ‘Good Afternoon Sir!’. And if it’s ‘Evening’, you have to say ‘Good Evening Sir!’.
Here ‘Morning’, ‘Afternoon’, and ‘Evening’ are conditions that need to be satisfied, and ‘Good Morning Sir!’, ‘Good Afternoon Sir!’, and ‘Good Evening Sir!’ are statements that you will perform on the fulfillment of the conditions.
If else in Python
In Python If Else conditions, there is a slight keyword difference as compared to other languages. Here we use elif in place of else if to check the mid conditions.
The elif keyword is Python’s way of saying “if the previous conditions were not true, then try this condition”. and the else keyword catches anything which isn’t caught by the preceding conditions.
Learn Python Basics
Python Conditions
Python supports the usual logical conditions from mathematics:
- Less than: a < b
- Greater than: a > b
- Equals: a == b
- Not Equals: a != b
- Less than or equal to: a <= b
- Greater than or equal to: a >= b
Python If Statement
tom_age = 30
brad_age = 25
if tom_age > brad_age:
print("Tom is elder than Brad")
As the given statement is satisfied, the further provided statement will execute
Python If with elif
tom_age = 30
brad_age = 30
if brad_age > tom_age:
print("Brad is elder than Tom")
elif brad_age == tom_age:
print("Tom and Brad are of same age")
Here the first condition is not met, the first provided statement task is ignored. The second condition is staisfied, hence the second statement is executed.
Python If with elif and else
tom_age = 30
brad_age = 25
if brad_age > tom_age:
print("Brad is elder than Tom")
elif brad_age == tom_age:
print("Tom and Brad are of same age")
else:
print("Tom is elder than Brad")
Here the first two conditions are false and not satisfied. Hence the provided statement tasks are ignored. Since the third condition is met, it will execute the further statement task.
Short Hand If Else in Python
Short hand means, if you have just one statement to execute, the code can be written in just one line.
a = 70
b = 50
print('A is greater') if a > b else print('B is greater')
You can practice Python on our online python compiler
More to read