JavaScript is one of the evergreen front-end script languages, that has been used to make the web experience better. In JavaScript, we have various in-built methods for different functionalities. In this post, we will talk about Confirm() method of JavaScript and its use case.
Confirm() method in JavaScript is used to show a pop-up dialog box with the given message. It is used to let the user confirm a message or reject it.
Just like the alert() method in javascript which is also used to show alert pop-up box in the top midsection of a PC screen or in the middle section of a smartphone screen. The pop-up will not go and will not allow you to do anything unless you press the ok button it.
The difference between the alert() method and the confirm() method in Javascript is that, in Confirm method, we have two buttons in the pop-up box, one for ok and another to cancel the pop-up. See the image.
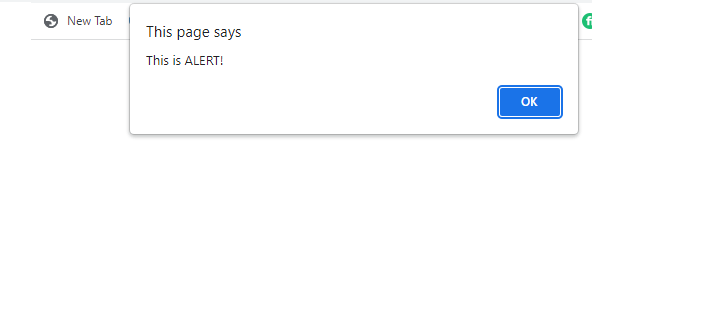
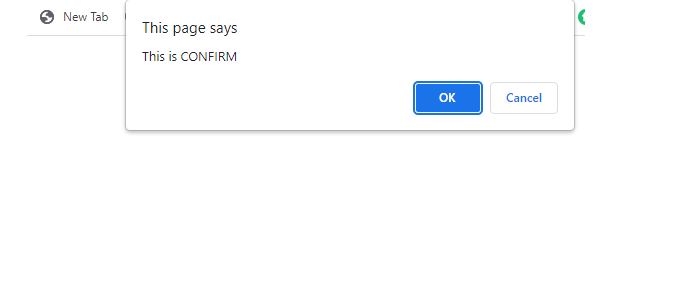
Syntax for Javascript Confirm and alert method
Javascript Alert() method
<script>
alert('This is ALERT!')
</script>
Javascript Confirm() method
<script>
confirm('This is CONFIRM')
</script>
We can also use Javascript confirm() with the window function like this:
<script>
window.confirm('This is CONFIRM')
</script>
We use Confirm() method in JavaScript in the situation where we need to create a pop-up with two values associated with it True and False.
The confirm() function returns true if a user has clicked on the OK button or returns false if clicked on the Cancel button. You can use the return value to process further. Let’s see the below code:
<script>
var userPreference;
if (confirm("Do you want to save changes?") == true) {
userPreference = "Data saved successfully!";
} else {
userPreference = "Save Cancelled!";
}
document.write(userPreference);
</script>
Let’s create a simple application for verifying userID using JavaScript prompt() and Confirm method().
In this application, we will ask for userID from the user using the pop-up prompt, then we will ask the user to confirm the userID. Whether the user confirms it or not, shows a respective message. Just paste the below code into a file and save it with .html file extension. Run the file to see the output.
<html>
<head>
<title>Javascript Confirm Method</title>
</head>
<body>
<div style="width:300px;height:50px;background-color:pink;margin:100px auto;" id="con"></div>
<script>
var id = prompt('Enter your userID');
if(confirm("Is the userID:" + " " + id + " right?")==true){
msg = 'You have confirmed your ID';
}else{
msg = 'You have not confirmed your ID';
}
document.getElementById('con').innerHTML = msg;
</script>
</body>
</html>