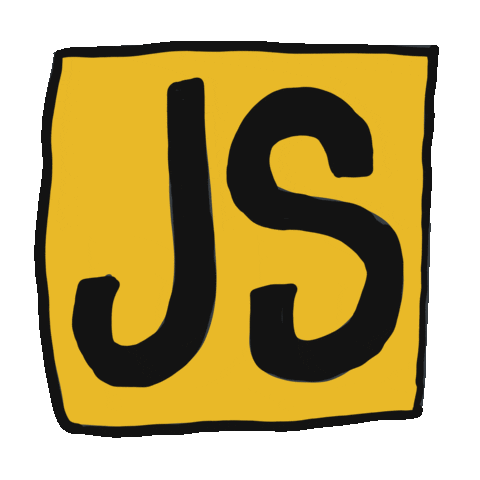
Exploring the “for each” Loop in JavaScript: A Comprehensive Guide
Introduction
As a student diving into the world of programming, understanding loops is crucial. Loops allow us to execute a set of instructions repeatedly, making our code more efficient and flexible. In JavaScript, one of the commonly used loops is the “for each” loop. In this article, we will explore the importance of loops in programming and delve into the intricacies of the “for each” loop in JavaScript.
Importance of Loops in Programming
Loops play a vital role in programming by enabling us to automate repetitive tasks. They allow us to iterate over collections of data, such as arrays or objects, and perform actions on each element without writing redundant code. By utilizing loops, we can streamline our code, make it more readable, and reduce the chances of errors.
Introduction to the “for each” Loop in JavaScript
The “for each” loop is designed specifically for iterating over arrays in JavaScript. It provides a concise and expressive way to access each element within an array and perform operations on them. While other loop constructs like the “for” loop have their own strengths, the “for each” loop simplifies the process of iterating over arrays.
Understanding the “for each” Loop in JavaScript
Basic Syntax and Structure
The basic syntax of the “for each” loop in JavaScript is as follows:
javascriptCopy codearray.forEach(function(currentValue, index, array) {
// Perform actions on currentValue
});
The “forEach” function takes a callback function as an argument, which will be executed for each element in the array. Within the callback function, you can access the current element being iterated over, its index, and the original array itself.
Iterating over Arrays with the “for each” Loop
Accessing Each Element in an Array
The “for each” loop simplifies accessing each element in an array. By utilizing the currentValue parameter in the callback function, you can directly access and manipulate the element at the current iteration.
Performing Actions on Each Element
Once you have access to each element, you can perform any desired actions, such as modifying the element, calculating values, or invoking functions based on specific conditions. This allows for easy data manipulation and transformation within an array.
Limitations of the “for each” Loop
Inability to Break or Skip Iterations
One limitation of the “for each” loop is the inability to break or skip iterations. Unlike the “for” loop, which allows for the use of “break” and “continue” statements, the “for each” loop continues iterating over all elements until it reaches the end.
Limited Support for Certain Data Types
Another limitation is that the “for each” loop is only applicable to arrays in JavaScript. It cannot be directly used with objects or other data structures. However, JavaScript provides alternative looping constructs for such scenarios.
Alternatives to the “for each” Loop
The “for…in” Loop
The “for…in” loop is an alternative to the “for each” loop when dealing with objects. It iterates over the enumerable properties of an object, allowing you to access and perform actions on each property.
Using Caution with Built-in Prototypes
When using the “for…in” loop, it’s important to exercise caution. It iterates over all enumerable properties, including properties inherited from the prototype chain. To avoid unexpected behavior, consider using the “hasOwnProperty” method to check if a property belongs to the object itself.
The “for…of” Loop
The “for…of” loop is another alternative loop construct introduced in ECMAScript 6 (ES6). It is particularly useful for iterating over iterable objects, including arrays, strings, maps, and sets.
Related posts
JavaScript Confirm() Method
JavaScript Array slice()
setTimeout Javascript