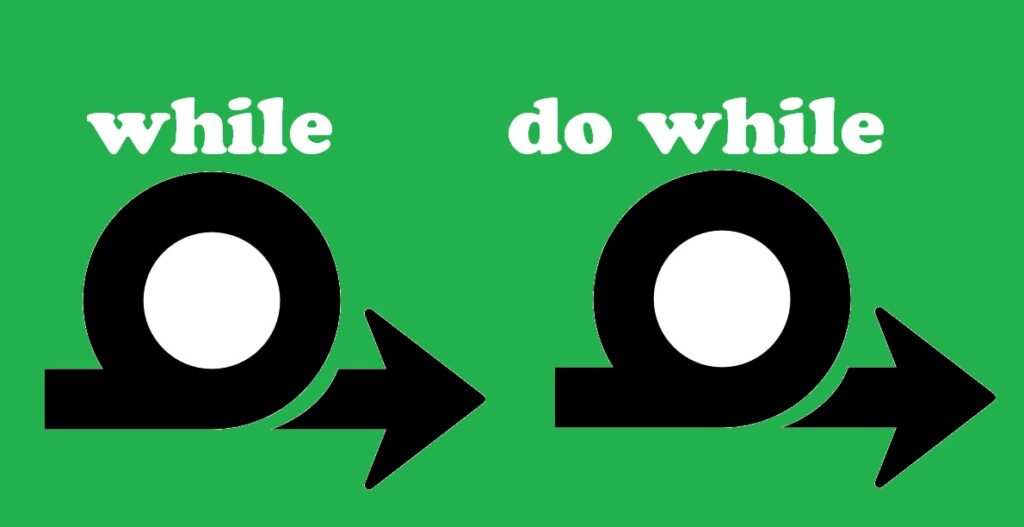
Loops in computer programming is a sequences of commands or instructions that are made for repeat until a required condition is met. You know humans get bored to repeat the same thing over and over and over. But machines can do the same task on repeat mode easily and quickly, even millions of times.
In any programming language, loops are some of the core important concepts, which is frequently used. In this post, we will talk about While and Do While loops in the C programming language.
While Loops in C
In the while loop, the code first checks the specified condition. If it is true, it will execute the further instruction, if the condition is false, it will end the whole instruction. Let’s see all these through examples:
#include <stdio.h>
int main() {
int i = 10;
while (i < 5) {
printf("%d\n", i);
i++;
}
return 0;
}
As you can see the condition (i<5) is not satisfied because we have set the value of ‘i’ as 10, the whole code will end up showing no result. Since the condition is not met, the while loop will not allow further code to execute.
#include <stdio.h>
int main() {
int i = 0;
while (i < 5) {
printf("%d\n", i);
i++;
}
return 0;
}
1
2
3
4
In the above code, since the condition (i<5) is True, the while loop will allow the further code to execute and it will perform the instruction as long as the condition remains in validity. As we can see above, after printing ‘4’, the value of ‘i’ increases to 5 and the condition (i<5) becomes False. So further execution of the code stops.
Do While Loop in C
Do While loop is an extension of the while loop with some uniqueness. It executes the code at least once doesn’t matter what the condition are.
This loop will execute the code block once, before checking if the condition is true, then it will repeat the loop as long as the condition is true.
This means, even if the condition is not met, the code will execute the instruction once, then it will decide to execute further depending on the condition satisfaction. Let’s see this by examples:
#include <stdio.h>
int main() {
int i = 10;
do {
printf("%d\n", i);
i++;
}
while (i < 5);
return 0;
}
As we can see, the condition (i<5) is False as we have set the value of ‘i’ as 10 initially, the code will execute once and will print the current value of ‘i’ which is 10.
#include <stdio.h>
int main() {
int i = 0;
do {
printf("%d\n", i);
i++;
}
while (i < 5);
return 0;
}
1
2
3
4
In the above code, since the condition (i<5) is True, the while loop will allow the further code to execute and it will perform the instruction as long as the condition remains in validity. Further, after printing ‘4’, the value of ‘i’ increases to 5 and the condition (i<5) becomes False. So further execution of the code stops.