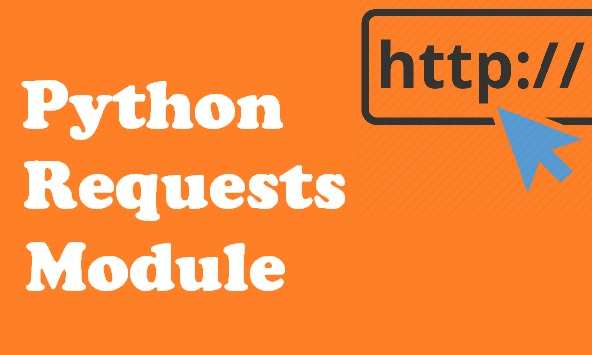
What is Python Request?
Python Requests is a module that allows you to deal with HTTP requests using Python. It is actually a simple API for making HTTP calls for sending and receiving data from the provided URL using various request methods.
There is already a built-in urllib library in Python that does the same job but the ‘request module’ is better and it simplifies the process of working with HTTP requests in Python by providing a higher-level interface.
With the requests module, you can send HTTP requests using Python and receive responses from servers. You can use requests to make all kinds of HTTP requests, including GET, POST, PUT, DELETE, and more.
On top of that, it also supports several other features, such as the ability to add HTTP headers, cookies, and other HTTP parameters to your requests. Learn Python Basics.
See an example:
import requests
response = requests.get('https://codespit.com')
print(response.text)
This will send a GET request to https://
codespit.com and print the response from the server.
Key Features of Python Requests Module?
Making HTTP Requests: The Requests module allows you to send various types of HTTP requests, such as GET, POST, PUT, DELETE, and more, by simply calling the relevant method (e.g., requests.get(), requests.post(), requests.put(), etc.). You can pass in parameters, headers, and other data as needed.
Authentication: The Requests module supports various types of authentication, including basic authentication, OAuth, and custom authentication methods. You can easily pass in authentication credentials as part of your request to access protected resources.
Handling Cookies and Sessions: The Requests module allows you to handle cookies and sessions easily. You can send cookies with your requests, and the module automatically handles session management and cookie persistence across requests, making it convenient for interacting with web applications that require session-based authentication.
Uploading and Downloading Files: The Requests module supports uploading and downloading files by simply passing in the file path or file-like objects as part of the request. You can easily upload files to a server or download files from a remote server using the module’s convenient file-handling methods.
SSL Verification: The Requests module allows you to easily control SSL certificate verification, allowing you to customize the security settings for your HTTP requests.
Handling Timeouts and Errors: The Requests module provides options for setting timeouts on requests, allowing you to control how long to wait for a response before timing out. It also has built-in error handling mechanisms that raise exceptions for common errors, such as connection errors, DNS resolution failures, and timeouts.
Different requests methods
There are various different request methods that a client can use when making a request to a server. Some of the most commons requests methods are:
GET | retrieves a representation of a resource |
HEAD | retrieves the headers for a representation of a resource |
POST | submits an entity to a resource, often causing a change in state or side effects on the server |
PUT | replaces a representation of the target resource with the request payload |
DELETE | deletes a representation of a resource |
CONNECT | establishes a tunnel to the server identified by the target resource |
OPTIONS | describes the communication options for the target resource |
TRACE | performs a message loop-back test along the path to the target resource |
PATCH | applies partial modifications to a resource |
How to install python requests module and use them?
First, you need to have Python installed on your local system. Once you have Python and by default pip installed in your system, you can use the code below to get the requests module.
pip install requests
This will download the requests package from the Python Package Index (PyPI) and install it on your system.
To use requests in your Python code, you will need to import it using the following import statement:
import requests
Then, you can use the various functions provided by the requests
module to make HTTP requests. For example, to send a GET request to a website, you can use the ‘requests.get()’ function as shown in the first code snippet above.
This will send a GET request to the website and store the response in a Response object. You can then access various properties of the response, such as the response body, headers, and status code, using the attributes of the Response object.
Here’s an example of how you can use the Python Requests module to make a simple GET request:
import requests
# Send a GET request to a URL
response = requests.get('https://www.example.com')
# Check the response status code
if response.status_code == 200:
# Print the response content
print(response.content)
else:
print('Error:', response.status_code)
Conclusion
The requests
module in Python is widely used to make HTTP/HTTPS requests to web servers and consume the responses. It provides a simple and elegant way to interact with web services and APIs, and is popular among developers for its ease of use and powerful features.
In general, the requests
module is useful whenever you need to send HTTP requests and handle the responses in Python. It simplifies the process of working with web services and APIs, and makes it easier to consume and manipulate data from the web.
You can read the full documentation for the requests
module here: https://docs.python-requests.org/en/latest/
Related:
Python Basics
List, Set, Tuple, and Dictionary in Python
Python Reverse List items
Python Round() Function
Python Multiline comment
Power in Python | Python pow()
Python range() Function
Square Root in Python
Python for i in range
Python Exception Handling